Embedded YouTube Player¶
Assuming the value of the field is a YouTube video ID, renders an embedded Youtube video when in read mode. Edit mode displays a boilerplate input element to update the field value.
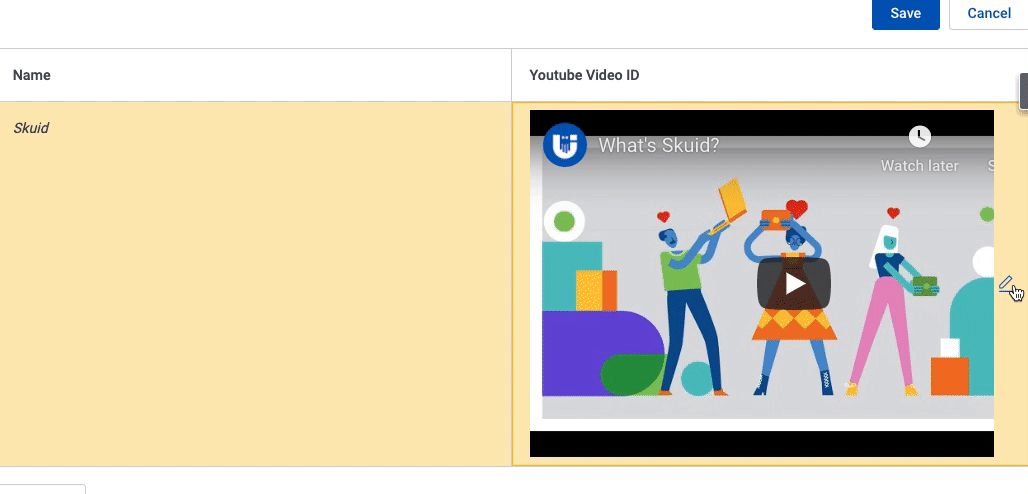
Example Code¶
Download the sample page for this field renderer here
, or see the sample code below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | const FieldRenderer = skuid.component.FieldRenderer;
return new FieldRenderer({
readModeStrategy: "virtual",
read: function (fieldComponent) {
let h = fieldComponent.h,
context = fieldComponent.cpi.getContext(),
{ field, model, row, value } = context;
return h("iframe",
{
width: "480",
height: "315",
src: `https://www.youtube.com/embed/${encodeURI(value)}`,
frameborder: "0",
allowfullscreen: "allowfullscreen",
}
);
},
editModeStrategy: "virtual",
edit: function (fieldComponent) {
let h = fieldComponent.h,
context = fieldComponent.cpi.getContext(),
{ field, model, row, value } = context;
return h("input",
{
value: value,
styles: {
width: "100%",
height: "32px",
padding: "0 8px",
},
oninput(event) {
model.updateRow(row,
{ [field.id]: event.target.value },
{ initiatorId: fieldComponent.id }
);
},
onblur(event) {
fieldComponent.cpi.onInputBlur();
},
}
);
},
});
|